This is my Graphics CPE 471 final project.
Overview
For my project I generated trees using L-Systems. An L-System is a parallel rewriting system and a formal grammar. They are used to describe plant cells and model plant growth processes. An L-system consists of an alphabet that can be used to recursively generate a string. The alphabet is made up of rules that can be translated into different geometric structures. An L-System begins with an axiom that consists of variables that represent other rules. Each iteration, the string grows by executing each rule.
Example L-System (from Wikipedia):
Lindenmayer's original L-system for modelling the growth of algae.
variables : A B
constants : none
axiom : A
rules : (A → AB), (B → A)
which produces:
n = 0 : A
n = 1 : AB
n = 2 : ABA
n = 3 : ABAAB
n = 4 : ABAABABA
n = 5 : ABAABABAABAAB
n = 6 : ABAABABAABAABABAABABA
n = 7 : ABAABABAABAABABAABABAABAABABAABAAB
Implementation
An L-System can have rules that define branching and growth sequences in a tree. Here are the rules for my L-Systems:
f : create branch
l : create leaf
C : fruit
[ and ] : define a set of local area/branch. The definitions of the area are put inside the [ and ]
+ and - : rotate the branch right/left around x-axis
^ and v : rotate the branch up/down around y-axis
<> : twist the branch left/right around z-axis
\ / : increment/decrement size of branch/leaf
{} : translate along x axis
() : translate along z axis
Here are the two algorithms for my project:
Algorithm 1:
axiom: fA
productionRuleA: ^f<<\Bl<<<<\Bl>>B>>>Bl>>>>>Bl
productionRuleB: [^^f>>><<<\lAC]
Algorithm 2 (Christmas Tree):
axiom: fffA
productionRuleA: ff[+++++++++fB][^^^+++++++++fB][^^^^^^+++++++++fB][^^^^^^^^^+++++++++fB][^^^^^^^^^^^^+++++++++fB][^^^^^^^^^^^^+++++++++fB]A
productionRuleB: [<<<\fB[<<\l][>>l][<<{{lO][>>}}l][<<<<\l][>>>>l]][>>>/fB[>>l][<<\lO][<<{{l][>>}}l][>>>>l][<<<<\l]]fB
The rules can be translated into transformation operations and applied to a hierarchical model where [ ] are essentially pushMatrix and popMatrix.
Result
Spring
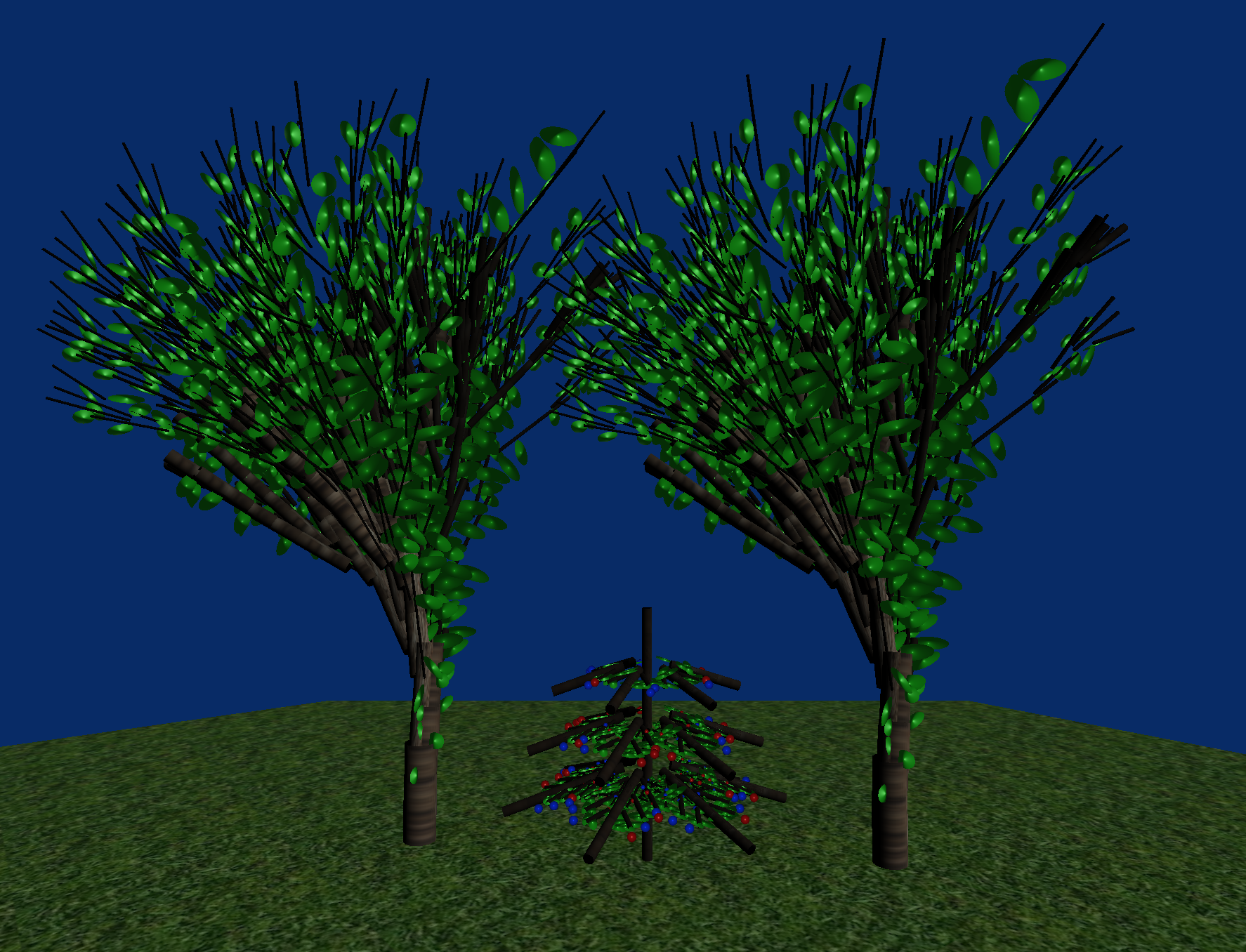
Summer
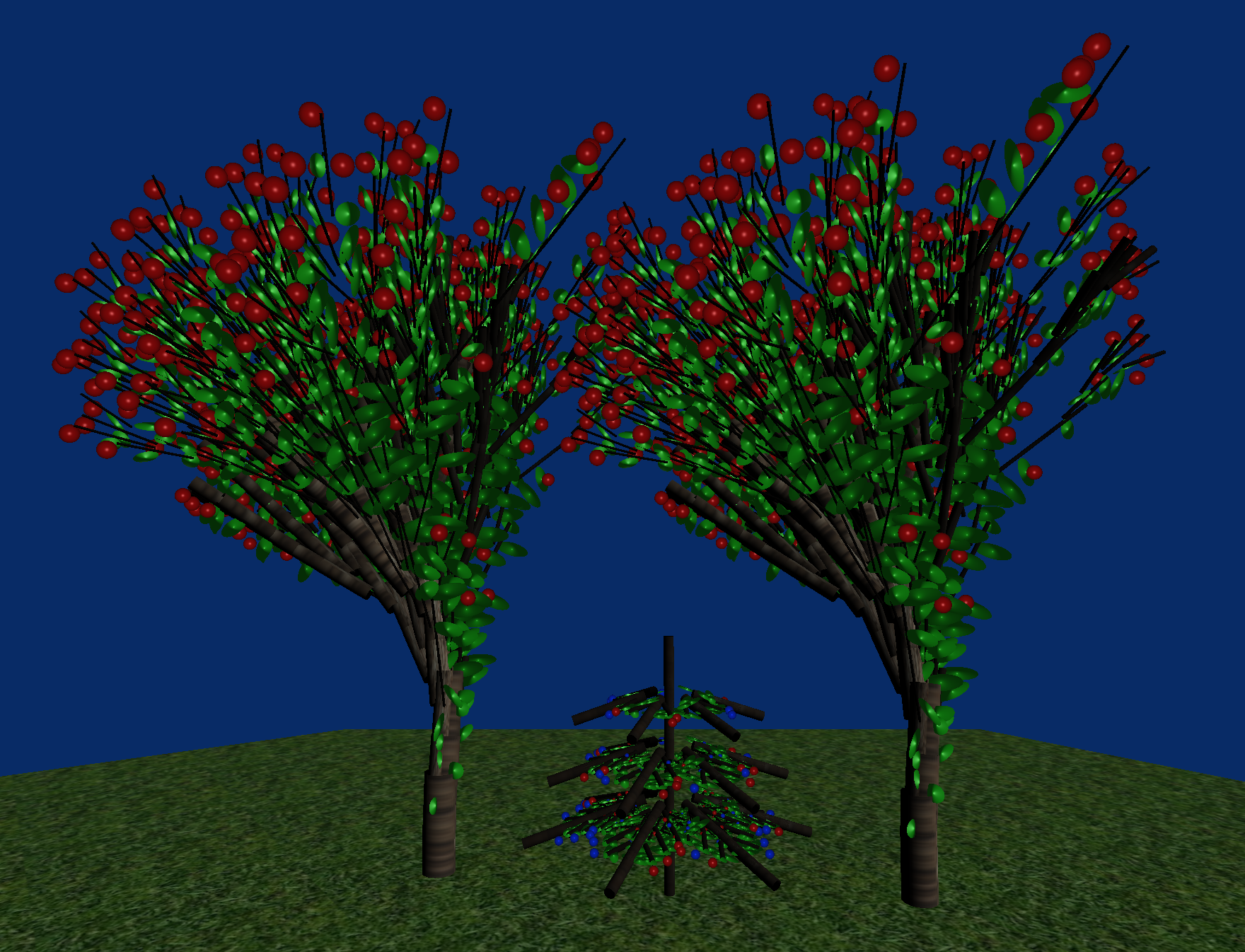
Fall
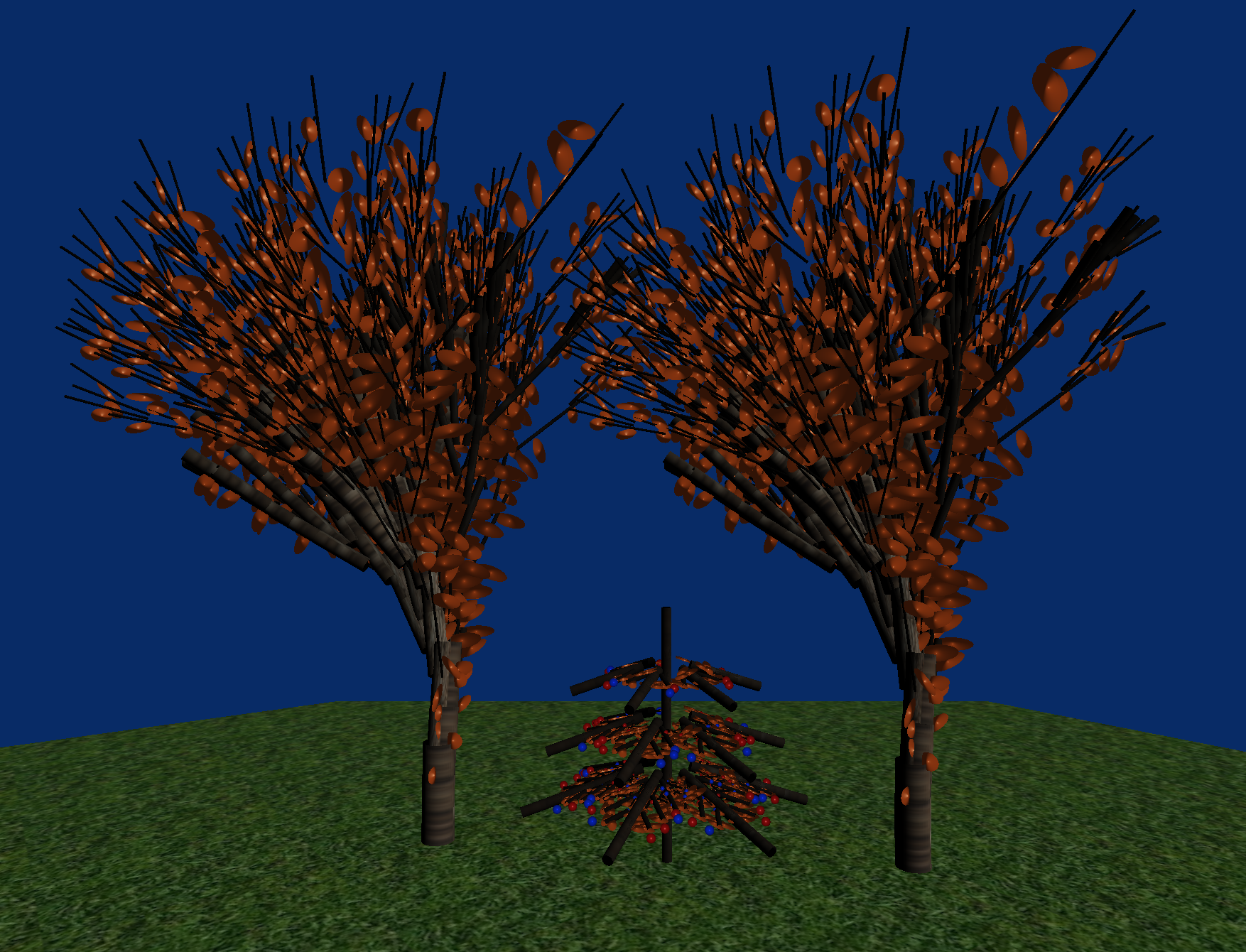
Winter
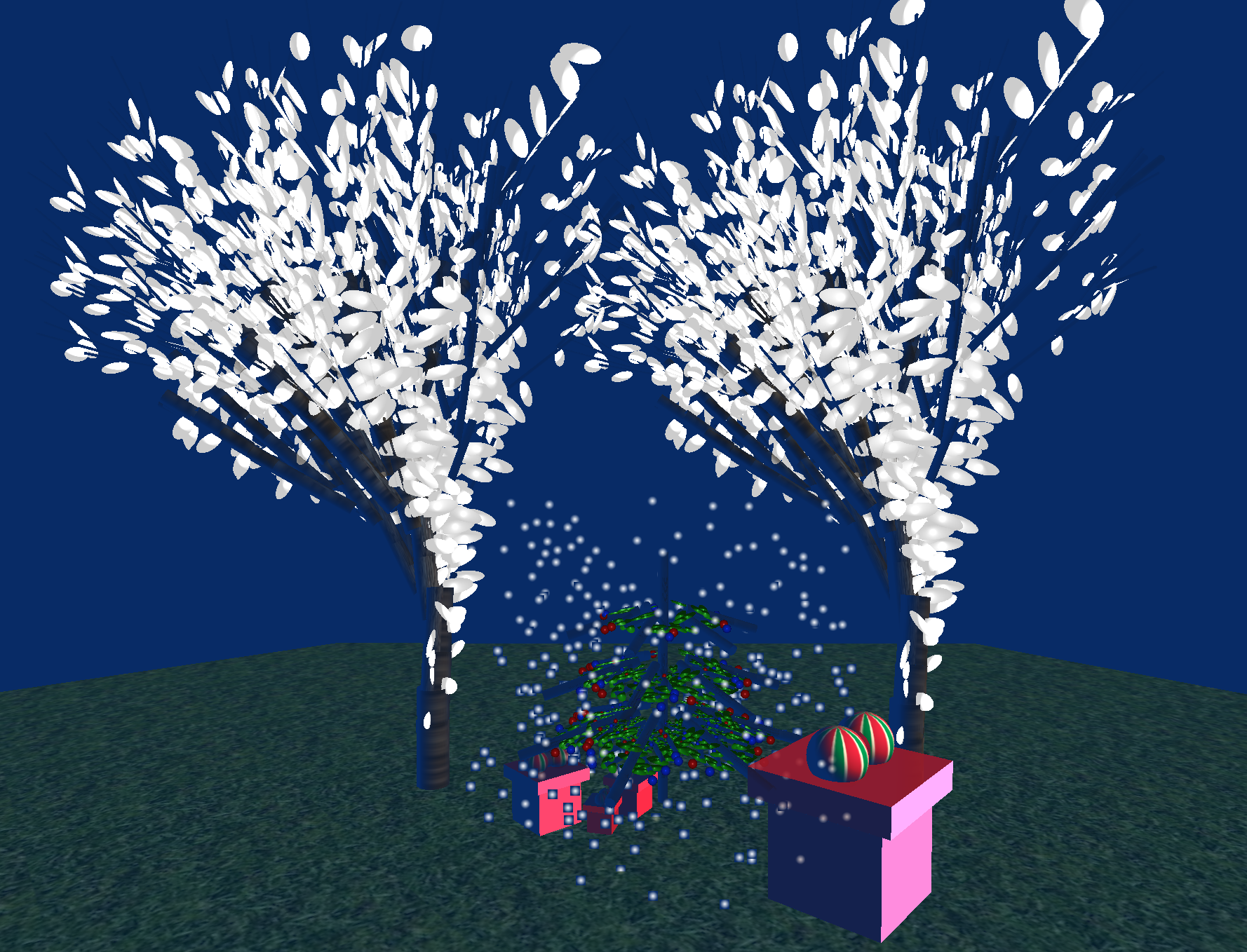
Tools used
The tools I used were OpenGL and the hierarchical modeling base code. I wrote my own function to recursively generate the production rules to generate the two trees. I used a cylinder object mesh to generate the trunks and scaled each trunk based on it's distance from the root. The leaves are scaled spheres that are textured with an image of a leaf. The particles are based on the particle base code given in class.