CPE 101 Laboratory 1
Due Date
- The end of week one; Friday 5:00pm
- You must turn in your work electronically on unix using the
handin command – instructions are provided below.
Objectives
- To login to your CSL and Unix accounts and change initial
passwords (if necessary).
- Familiarization with the specific programming environment and
tools.
- Familiarization with the general computing environment.
- Successful enter a C program (provided), compile it, and turn it
in electronically.
- Learn the syntax of simple C programs.
- Practice using the handin utility to turn in program source
electronically.
Resources
Ground Rules
This is an individual activity. You may get assistance from your
peers but you should complete all the steps yourself.
Part 1: The Computing Environment
This quarter you will be using two different computing platforms:
- The workstations in the Computer Systems Labs (CSL) computers
running Linux (specifically, CentOS).
- The CSc department's Unix server on a remote computer with the
name of
unix1.csc.calpoly.edu
On the unix1 server you will be
using a text editor and the GNU C compiler, gcc, to develop,
compile, and test your programs. Assuming you have an internet
connection at home you can also access hornet from your own personal
computer (PC, Mac, Linux, …). Note: If you login from off-campus
you will need to use unix1.csc.calpoly.edu as the host name
when connecting. This can be a convenient way to access your work
from home and school – simply make sure that you most current work is
on unix1 and you’ll be able to access it remotely.
Another important detail to know and understand is that all of the
workstations in the CSL (rooms 235, 301, 302, and 303) share a
central file server. Your accounts are set up with the home
directory mapped to this file server. You’ll be able to login
to any workstation in these rooms and have access to your
files.
The Linux Workstations
These directions apply to any workstation in 14-301,302,303,235, etc.
The machines are configured to dual-boot Windows 7 and CentOS
Linux. We will be using Linux in this course.
- Find a machine displaying the Linux login dialog box.
- You login credentials are the same as for your Cal Poly portal
access.
- If it refuses to authenticate you, check the Caps Lock key.
Also,
if you weren't enrolled on day one there will be a delay in getting
your account created. See the instructor if you need assistance.
- Once logged in you'll see the Linux desktop, which uses a
familiar
"Windows, Icons, Menus, and Pointers" (WIMP) interface.
- Explore the Applications menu to discover what programs are
installed
on the computer. You should find an Office suite, graphics
programs, a web browser, music player, games, and other common desktop
applications.
- The Places menu opens a file system browser. Observe
what
files and folders already exist in your account.
- Create a folder to contain your documents for this
course.
A suggested folder name is "cpe101".
- From the Applications menu, select Accessories and then Text
Editor. This launches a simple text editing program. Enter
the name of your favorite reptile and then save the file in your "My
Documents" folder with the name "reptile.txt".
Creating a program
Launch the Text Editor. Copy and paste the following eight
lines into a new text document.
#include <stdio.h>
/* Print a simple message to the screen. */
int main(void)
{
printf("Greetings, Earthling.\n");
return 0;
}
Save the file in your home folder with the name "greetings.c".
(Don't type the quotation marks).
You should be able to verify the size and date created of the file from
the File Browser.
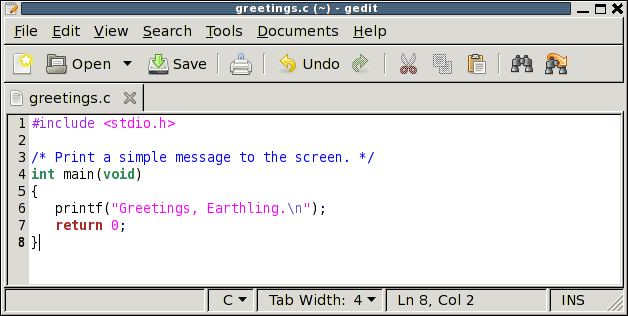
Compiling a program
From the applications meu, select Accessories, and then System Tools,
then Terminal. When launched, it
will open a small window where you interact with the system via a
command line interface (CLI). The CLI is the traditional way to
communicate with Unix where you type commands to tell the computer what
to do. That is faster and more powerful than a graphical interface, but
requires recalling or looking up the needed commands rather than
finding them in a menu. While it may appear foreign and
intimidating at first, anyone who can manage typing, backspacing, and
cutting and pasting can manage a CLI.
When the terminal is first opened, you will observe white text on a
black background by default. Your username and the workstation
name is displayed, followed by a blinking cursor. By
default, the terminal opens in your home directory.
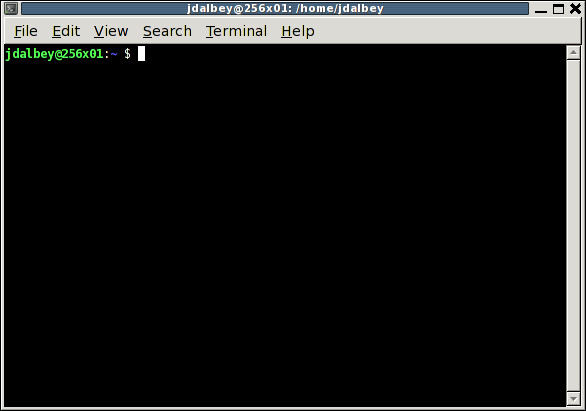
Type: ls
This displays the names of all the files in the current
directory. You should see the name "greetings.c" that you saved
in the previous step.
Type: gcc greetings.c
What does this mean? GCC is the name of the C compiler we will
use throughout this course. To use this compiler, we type the
command “gcc”. The compiler turns our C code into something the
machine can execute. We provide the filename "greetings.c" as part of
the command to tell the compiler the name of the file that contains our
program. We saved the program as “greetings.c” in the previous
step. You can think of "greetings.c" as the input data the
compiler will process.
If you’ve typed everything correctly up to this point, you shouldn't
see any output when you run gcc, only the prompt. That's because
when the compiler completes its job without an errors it produces no
feedback. It's probably not the best way to design a user
interface, but that's how Unix does it. You get no feedback
if you do things right, but errors if you make a mistake. If you
see any warnings or errors when you run this command, go back and
double check that you've typed everything exactly as shown. When
I run it, it looks like this:
jdalbey@302-01:~$ gcc greetings.c
jdalbey@302-01:~$
The results from running the compiler is a file containing binary
machine instructions (an executable) and by default this file is named
"a.out." There's no rhyme or reason for this odd
name. Later you can use the File Browser to rename it
something more meaningful.
If the previous step completed successfully (gcc doesn't display any
output), you can run your program.
Type: ./a.out
(That's a period, a forward slash, then a.out)
The greeting message should be displayed. If none of this is
working for you, ask for assistance. When I run it, I get:
jdalbey@302-01:~$ ./a.out
Greetings, Earthling.
jdalbey@302-01:~$
Congratulations, you have just compiled and run your first C program!
The Unix Server
The CSc department's Unix server is a remote computer with no direct
physical access. Its address on the internet is
unix1.csc.calpoly.edu.
You can create and compile programs on the server, just like on the
workstation. You will also be using the server to submit your
work.
Follow the directions in
Submitting
your work to submit your "greetings.c" file.
Part 3: Learning syntax of C programs
In this activity you will learn about the detailed syntax of a correct
C programs by entering the source code for a program from a written
listing.
- Obtain the handout from the instructor that contains the source
code for the ConePaint program.
- Study the listing to understand the purpose of the program.
Starting at the comment that says "Convert dimensions to feet" manually
perform the calculations for each statement shown in the
program. (You may use a calculator). You should be
able to predict the exact results that will be displayed by the
finished program. Write your prediction in your lab
notebook. (Feel free to refer to Chapter 2 in the textbook or
consult with a peer or the instructor. If this step seems
overwhelming to you at this point, it's okay to skip it and continue to
step 3.)
- Open the Text Editor and type in the text
from the listing exactly as it is shown on the handout. Save the
program as conepaint.c
- Compile the program. If you have entered the text without
any mistakes, it will compile without errors. If gcc reports
warnings or errors, you must examine the source code you entered and
compare it to the listing to determine where you made a mistake.
Here's a
list of common error messages and
solutions.
(Tip: In the Text Editor, pull down the Edit menu, select "Preferences",
and check the box next to "Display line numbers".)
- Execute the program. Compare the actual output to your
predictions. If there is a discrepancy, determine if you
calculated wrong or if there is an error in the program. Record in your
lab notebook how your resolved the discrepancy.
- Locate the statement in the program
#define kRedPrice 10
In your notebook, calculate the results if you were to change
the "10" to "20".
Then make that change to the program and execute it. Compare the
actual output to your
predictions.
- There is nothing to submit for this part of the lab.
Part 4: Running interactive programs
- Examine the source text for the Number
Juggling
Program.
- In a manner similar to part 3, predict the results from executing
the program. The important difference in this program is that the
user must provide input data. Use your own birthdate and age as
input values. Predict the result that the program will
produce. Write your prediction in your notebook.
- Open the Text Editor and and Copy-and-Paste the
text from the listing. Save the program as numberjuggle.c
- Compile the program. It should compile without
errors.
- Execute the program. When the program displays the prompt,
provide your input data. Observe the actual output that is
produced. Compare the actual output to your
predictions. If there is a discrepancy, determine if you
calculated
wrong or if there is an error in the program. Record in your lab
notebook how your resolved the discrepancy.
- Here's what a sample execution looks like, with the user input
underlined:
Here is a clever number juggling trick.
Enter the year of your birth (positive 4-digit integer): 1930
Enter your current age (positive integer): 80
... and after juggling the numbers, the result is: 1930.83
Isn't that amazing?
- There is nothing to submit for this part of the lab.
Part 5: Finding Defects
- Read the header comments to determine the purpose of the Cable Revenue Program.
- The source code has several defects that have been inserted by
the instructor. Study the source code and try to identify the
mistakes. Note them in your lab notebook.
- Open the Text Editor and Copy-and-Paste the
text from the listing. Save the program as cablerevenue.c
- Compile the program. Several errors will be reported.
- Attempt to fix the errors. In some cases the error message
is very obvious and you will see immediately what needs to be
corrected. In other cases, the error message is vague or
confusing. You might find this list of common
error
messages helpful. Write each error message and the correction
which fixes it in your lab notebook. If you are stuck, you may
get assistance from your peers or the instructor.
- Execute the program. When the program displays the prompt,
provide the sample data of 3 installations and 15 yards of
cable. Observe the actual output that is
produced. Compare the actual output to your
predictions. If there is a discrepancy, determine if you
calculated
wrong or if there is an error in the program. Resolve the discrepancy
and describe it in your lab
notebook.
Part 6: Submit your completed program
Submit your completed Cable Revenue Program electronically, using the
procedure described in the Submitting Your Work tutorial above.
- Use the handin
command below:
handin graderjd Lab01
cablerevenue.c
Part 7: Setting up your home computer (optional)
If you have a home computer you will probably want to configure it so
you can work on your coursework at home.
Here is a tutorial on
developing programs remotely on the CSc Unix
server.
The instructor
recommends using Linux (or Mac). If you have Windows there are
several alternatives:
Install PuTTY
and connect to unix1 remotely.
Run the Windows
installer for Ubuntu Desktop.
Run Ubuntu from a Live-DVD.
Configure your machine to dual-boot
Windows and Linux.
Extra Credit
Vi intro
Play Vim Adventures
Vi project