2.8 Script Editing
Scripts can be created that will allow the prototype to perform some actions or calculations. The scripts will follow the JAVA syntax (For more information on Java please see Sun's Website. To add functionality to the prototype, scripts can be executed when an action is performed on a component or sensitized object. Please refer to Section 2.7 for information on how to assign a script to an object, or continue reading to learn about edititing scripts.
2.8.1 Using the Script Editor
The Script Editor is the only method of editing scripts. The Script Editor is automatically invoked whenever the user chooses to edit a script for a specific action, so the user will never have to concern themself with naming script files or method names. The below prototype or some derivation of it (see Script Naming Conventions) will automatically appear when the Script Editor opens. Once the user is through editing the script, they have the choice of either the 'Cancel' or 'OK' buttons which are located at the bottom of the Script Editor window. The 'OK' button will, with no visibile output to the user, save the script in the correct location and assign it to be executed when the appropriate action is performed and then close the Script Editor window and return the user to their previous location. The 'Cancel' button will close the Script Editor button without saving the script.
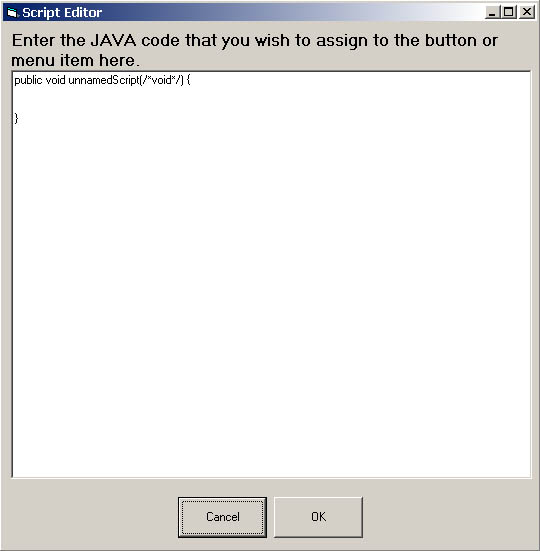
Figure 23: Initial Script Editor Window
2.8.2 Rules for creating scripts.
-There must be exactly one public void function with no parameters per script.
-The public void function will be given a default name based upon what calls it. This name can be changed by the user
-There may be from 0 to N private functions per script. These may be void or function returning and are allowed to have parameters.
2.8.3 Detail Commands availible
Every kind of object has several default functions or actions that can be performed upon them through the scripting language. The list below contains the built in commands that are availible organized by type of objects. New commands can be built through the use of scripts.
Frame
-Open()
-Close()
-Minimize()
-Maximize()
-Visible()
-Invisible()
-bringToFront()
Text Box
-putText("TextString")
-Visible()
-Invisible()
Menu Bar
-Visible()
-Invisible()
Image
-Visible()
-Invisible()
Check Box
-Check()
-Uncheck()
Vertical Scroll Bar
-Visible()
-Invisible()
Horizontal Scroll Bar
-Visible()
-Invisible()
Radio Button
-Select()
-Unselect()
Button
-Visible()
-Invisible()
-leftMouseUp()
-leftMouseDown()
-rightMouseUp()
-rightMouseDown()
-doubleMouseClick()
-mouseEnter()
-mouseLeave()
-keyPress("CTRL-A")
Drawing Object
-Visible()
-Invisible()
Sensitized Drawing Object
-Visible()
-Invisible()
-leftMouseUp()
-leftMouseDown()
-rightMouseUp()
-rightMouseDown()
-doubleMouseClick()
-mouseEnter()
-mouseLeave()
-keyPress("CTRL-A")
2.8.4 Example Script
The scripting language will follow the syntax rules of the Java programming language, but will have built into it all of the commands that are availible as buttons to the user. Such buttons/functions will include Close Window, Maximize Window, Minimize window and Bring Window to front. Other functions that will also be availible in the scripting language will be commands to Open a window or to make a hidden window Visible. All sensetized objects and component buttons that are in the project will also be availble to the user to invoke as functions. A script file appears below.
This assumes that there already exists an open Frame named 'Frame1' and that another Frame 'Frame2' has been designed, but is not visible. The user has clicked the mouse on a button in 'Frame1' named 'Button1'. The purpose of this script is to close 'Frame1' and then open 'Frame2' and minimize it and then cause a sensetized object ('obj2') in 'Frame2' to recieve a right mouse click and execute the associated script or action.
======================================
public void Button1_rightMouseClick(/*void*/) {
Frame1.Close();
Frame2.Open();
Frame2.obj2.rightMouseClick();
Frame2.Maximize();
}
======================================
The following Figures illustrate the different steps of the above script being executed. The first figure shows the program before any input by the user has taken place. The second figure shows immediately after the button has been clicked. Frame1 has been closed, and then Frame2 is opened. Then in the third figure is shown the results of the button in Frame2 being clicked. Then in the fourth and final frame, Frame2 is maximized.
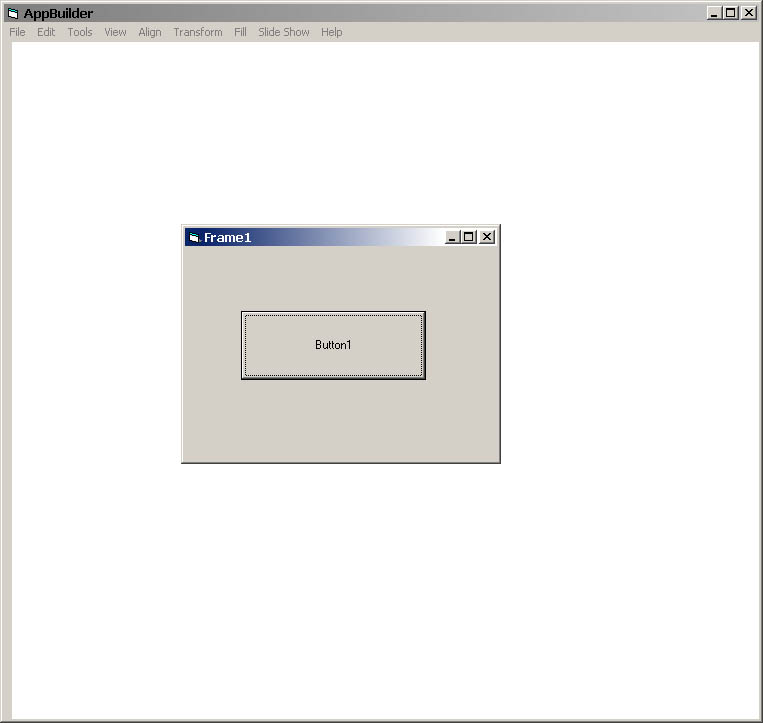
Figure 24: Screen before Button1 is pressed.
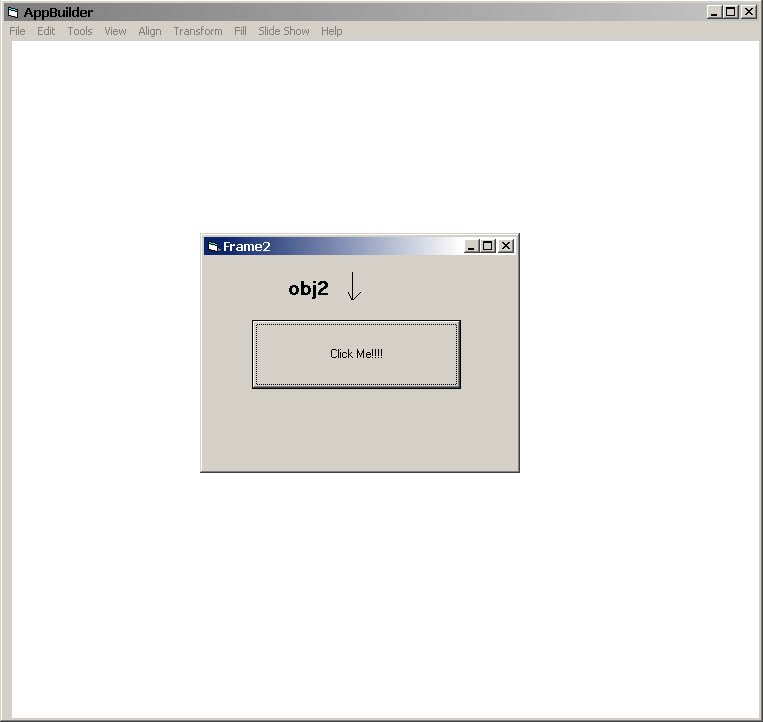
Figure 25: Screen after first and second line of code in above script executed.
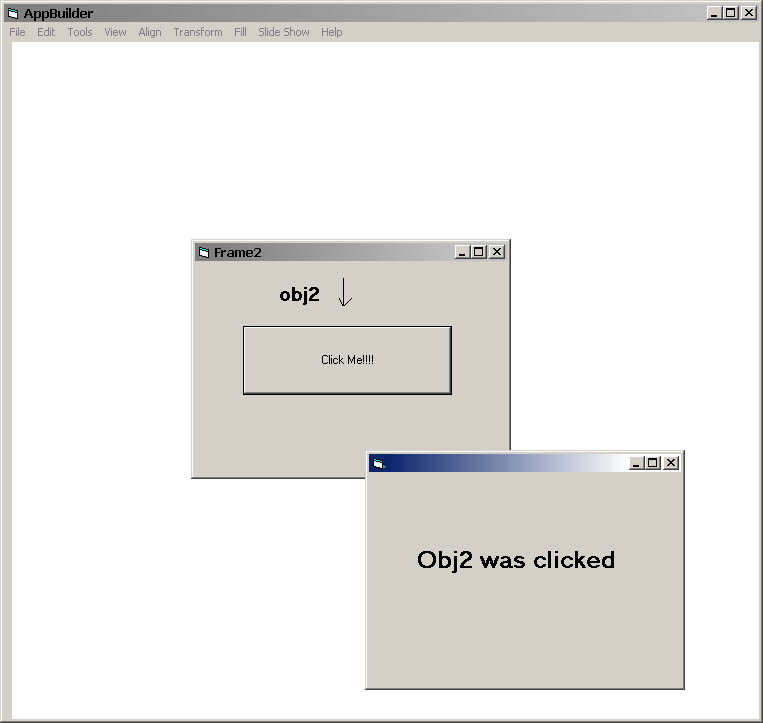
Figure 26: Screen after third line of code in above script executed.
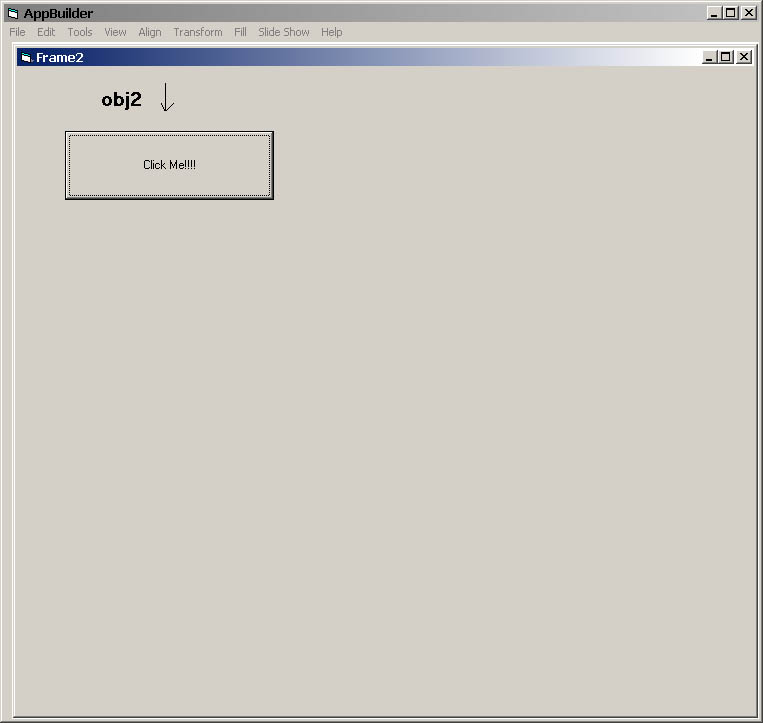
Figure 27: Screen after entire script has executed.
2.8.5 Script Naming Conventions
When the Script Editor is loaded, it will provide a template for a public void function with a name that is the same as the name of the object that the script is being assigned to. Then the function name will have an '_' followed by a string identifier of the type of action that will invoke that specific function. For example if a particular script is being assigned to a right mouse click on a button then the string identifier would be rightMouseClick. These are all concatanated together to form the function name, which in this example would be 'button1_rightMouseClick()'.
These words and images are property of James C. Irwin, jcirwin@calpoly.edu
05/1/2002